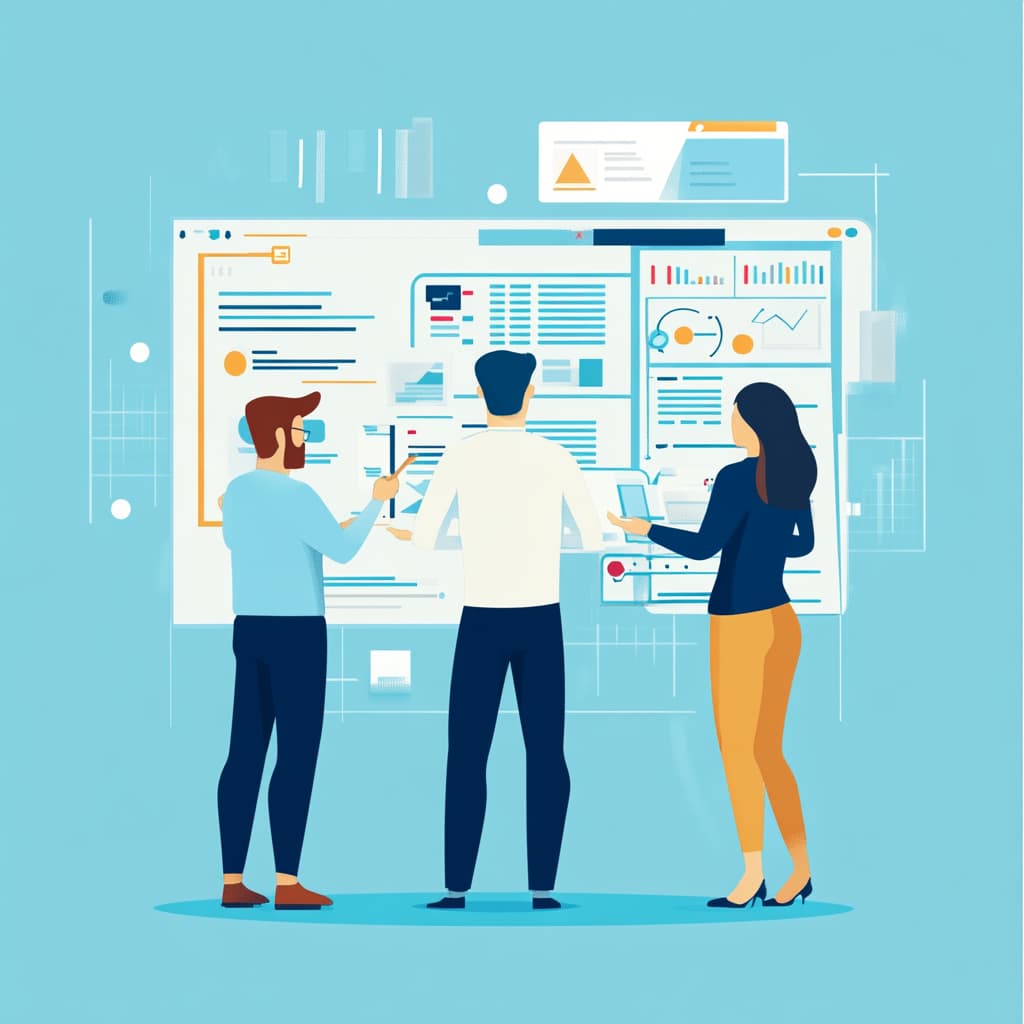
Code review is an essential part of modern software development. It improves code quality, ensures consistency, encourages knowledge sharing, and reduces bugs before they reach production. But for many developers, having their code reviewed can feel daunting—especially when deadlines loom and feedback can be highly detailed (and sometimes critical).
Preparing your code for review doesn’t just make the process smoother—it shows respect for your peers’ time and helps you grow as a developer. In this article, we’ll break down how to properly prepare your code for review, focusing on three key areas: code structure, documentation, and common anti-patterns to avoid.
Why Preparing for Code Review Matters
Before diving into best practices, let’s quickly highlight why good preparation is so important:
- It increases the chances of your code being approved quickly.
- Reviewers can focus on logic and architecture instead of chasing formatting issues.
- You reduce back-and-forth comments on avoidable mistakes.
- You demonstrate professionalism and accountability.
A well-prepared pull request reflects a well-organized mindset—something every engineering team appreciates.
1. Structure: Organize Your Code Logically
Clean and logical structure makes your code easier to read and understand. Even if your code works perfectly, reviewers may reject it if it’s messy or inconsistent with project conventions.
Follow the project’s style guide.
Most teams use a coding standard (like PEP8 for Python, Google Java Style Guide, or Airbnb’s JavaScript guide). Adhering to these standards saves reviewers from having to correct minor issues like indentation or naming.
Keep functions short and single-purpose.
Long, multi-functional methods are hard to test and understand. Aim for modular code with clearly defined responsibilities. If a method is doing more than one thing, split it up.
Use meaningful names.
Avoid vague names like data1 or helper. Instead, choose names that clearly describe purpose, like userList, sanitizeInput, or fetchCustomerData.
Group related logic together.
Don’t scatter related code across different files or layers. If you’re implementing a new feature, place the relevant logic, models, and configurations in coherent places.
Remove commented-out code.
Dead code is noise. If you no longer need it, remove it. If it might be needed later, version control has your back.
Refactor before review.
Don’t use the review process to clean up obvious code smells. Reviewers should assess logic and architecture—not clean up technical debt that could’ve been fixed earlier.
2. Documentation: Explain What, Why, and How
Good documentation helps reviewers understand not just what the code does, but why it was written a certain way. It also helps future developers (including future you) navigate the code months later.
Write a clear pull request description.
Start by explaining the purpose of the change:
- What problem does it solve?
- What new behavior does it introduce?
- Are there any dependencies or migration steps?
- How was it tested?
Bonus points for adding screenshots, links to Jira tickets, or diagrams that explain complex logic.
Use inline comments where necessary.
If a block of code involves a non-obvious algorithm, tradeoff, or hack, explain it with a brief comment. Avoid over-commenting simple logic, but do annotate when the code’s intent may not be clear.
Update external documentation.
If your change affects the public API, deployment process, configuration files, or user-facing behavior, update the relevant docs before the review. This prevents bottlenecks later.
Document assumptions and limitations.
If your code relies on certain conditions (e.g., “Assumes input data is always sorted”), spell them out. Reviewers can then validate those assumptions—or challenge them.
3. Anti-Patterns to Avoid
No matter how good your logic is, certain patterns will raise red flags during code review. Being aware of these common pitfalls can help you avoid unnecessary rework.
Hardcoding values.
Avoid magic numbers, hardcoded file paths, or API keys. Use constants, environment variables, or configuration files instead.
Copy-paste coding.
Duplicating code increases maintenance overhead and introduces potential bugs. If you’re copying logic from elsewhere, consider abstracting it into a reusable function.
Overengineering.
Don’t design for every possible future use case. Keep your code as simple as possible for the current requirement. Premature abstraction can make code harder to maintain.
Insufficient error handling.
Always consider how your code behaves under failure conditions—invalid input, timeouts, or system errors. Use try/catch blocks appropriately and provide meaningful error messages.
Deeply nested logic.
Multiple levels of nested conditionals make code hard to read and test. Aim to simplify or refactor deeply nested blocks into smaller helper methods.
Poor test coverage.
If your code isn’t tested, expect pushback. Write unit tests for all critical paths and edge cases. Include integration tests if your change affects multiple components.
Overuse of comments instead of refactoring.
If you find yourself needing to explain too much in comments, it’s a sign the code could be simplified. Well-written code should be mostly self-explanatory.
Bonus: Run Your Own Mini Review
Before submitting your pull request, take 10–15 minutes to review your own code from a reviewer’s perspective:
- Would someone unfamiliar with this feature understand what’s happening?
- Are there parts that look suspicious or unnecessarily complex?
- Did I leave behind any debug logs or temporary commits?
- Are all tests passing? Did I test edge cases?
Self-review shows maturity as a developer and reduces friction in team workflows.
Conclusion
Code reviews are an opportunity—not a hurdle. When you prepare your code well, you not only make your reviewer’s life easier, but you also improve your own craftsmanship. Well-structured code, thoughtful documentation, and awareness of common anti-patterns reflect the habits of a professional developer.
In fast-paced development teams, quality is everyone’s responsibility. Whether you’re a junior writing your first PR or a senior leading a critical refactor, the way you prepare your code sets the tone for collaboration.
So next time you push a branch, take a breath, tidy things up, and ask yourself: “Would I enjoy reviewing this code?” If the answer is yes—you’re already one step ahead.